Yummy CakePHP functions you should know about!
Posted on 4/6/06 by Felix Geisendörfer
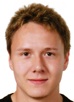
Deprecated post
The authors of this post have marked it as deprecated. This means the information displayed is most likely outdated, inaccurate, boring or a combination of all three.
Policy: We never delete deprecated posts, but they are not listed in our categories or show up in the search anymore.
Comments: You can continue to leave comments on this post, but please consult Google or our search first if you want to get an answer ; ).
One of the fun things when working with CakePHP has always been browsing through the core code. It truly is a masterpiece of PHP programming art, and you'll almost always find something interesting to use in your current projects. So this post will give you a little insight into some of those seldom used CakePHP features I've come across.
CakePHP's build in data cache
Function: cache($path, $data = null, $expires = '+1 day', $target = 'cache')
Purpose: Allow people to store temporary data in the app/tmp/cache folder. (And inside app/webroot as well)
The cache function is an easy way to store the results of server-heavy requests for an hour or two so the performance won't suffer. I found it very useful when working with Web Services. So here is a simple way to use this function (out of my Google Analytics Model):
$profilesData = cache($cachePath, null, '+2 hours');
if (empty($profilesData))
$profilesData = cache($cachePath, $this->httpGet($url));
If you need to cache objects, you can use the serialize / unserialize function of php. If you need to cache resized images you can set $target to 'public' and things will get stored to the webroot. All in all a very neat function ; ). One thing I created myself when working with it, was a function that would generate a unique $path based on the paramaters of the function. That way you can make sure the data you cache is only cached for one specific set of params:
{
$args = func_get_args();
array_shift($args);
$hashSource = null;
foreach ($args as $arg)
{
$hashSource = $hashSource . serialize($arg);
}
return md5($hashSource).$ext;
}
Now you can pass all parameters you want to the function and it will return a unique cache path based on the md5 fingerprint of the parameters. It'll work with Strings, Numbers, Arrays, objects and everything else that can be serialized.
Working with Trees in CakePHP
Function: Model::findAllThreaded($conditions = null, $fields = null, $sort = null)
Purpose: Return an array of nested Model Items based on their parent_id field.
If you know how to work with Model:findAll() you also know how to use this function. The only difference is, that this function expects your model (table) to have a field called parent_id where relations betweens items are stored. Based on that a nested array is returned.
CakePHP's build in debug() function
Function: debug($var = false, $showHtml = false)
Purpose: Output the data of any object or variable as long as DEBUG > 0.
If you have to use debug statements in your app in order to check results here and there, this function is the way to go. It basically does a print_r() on your data and puts it inside <pre> tags. Now when your application becomes stable you simply set DEBUG to 0 inside of app/config/core.php and all your debug() statements become deactivated.
Read & Write files
Read Function: file_get_contents($fileName, $useIncludePath = false)
Write Function: file_put_contents($fileName, $data)
Purpose: Simple access to read & write for files.
I think those two functions are php5 functions that cakephp creates for you if they are not available when you use php4. The functions themselfs are pretty self-explanatory since all they do is to read and write data from / to files.
Recursive chmod
Function: chmodr($path, $mode = 0755)
Purpose: Allows you to recursivly chmod an entire directory.
This function expects you to provide a $path and and chmod $mode, then it recursivley sets this $mode to all files, inside this directory recursivley. Useful when you do a lot with files in your app.
Take the best out of scaffolding
Function: Controller::generateFieldNames($data = null, $doCreateOptions = true)
Purpose: Generates an array that can be used to create a scaffolded interface based on your key model in the controller.
This is one of the *most* RAD functions in CakePHP I think. You usally use it like this in order to create a scaffold-like interface (only that you have full control over it):
$this->set('post', $post);
And inside your view you do somethings like this:
<?php echo $form->generateSubmitDiv('Create Post'); ?>
</form>
This will build you an interface based on the fields in your table that's a lot like the scaffolding one. If you need to customize it (which is why you do this instead of scaffolding), just take a look at the $post array created by generateFieldNames. Changing things around in there can help, as well as to unset() certain fields of it.
And there are more ...
Those are just a couple functions I've used before or found inside the CakePHP core. I know that there are tons of others, and that's why I would encourage everybody to go out for some adventures inside the code of the framework you work with - it'll be an educational experience.
I hope some of those functions will be helpful for you in future ; ).
--Felix aka the_undefined
You can skip to the end and add a comment.
Well, this looks great! I am trying to use the generateFieldNames stuff you mentioned. One thing I'm not so sure of is how exactly you can customize it. I guess you can customize all around the form, but not the form itself, right?
Hi nate, I assume your not the cakephp-devloper nate, right?
Anyway, you can use unset() to remove certain fields from beeing turned into an interface. You can manipulate the array items to change their types (text->dropdown, etc.). Now if you have to insert stuff in between or change the html generated you'll need buffer the output of the FormHelper::generateFields() function and use some regex on it to change/insert stuff. You might also be able to do that by adding a dummy value to the array and to replace the outputs for it (haven't tried that yet).
Normally I found CSS together with unset() good enough for customizing the interface to fit all my needs. If you really don't get output buffering and regex working for you I suggest you just copy the output of the generateFields() function directly into your view and custimize it there. Because you should only use generateFields for simple interfaces that go with the cakephp scaffolding kind of way. Otherwise you should implement your own forms.
Hope that helps, --Felix
[...] Yummy CakePHP functions you should know about!: "One of the fun things when working with CakePHP has always been browsing through the core code. It truly is a masterpiece of PHP programming art, and you'll almost always find something interesting to use in your current projects. So this post will give you a little insight into some of those seldom used CakePHP features I've come across. [...]
hi,
I am new to cake php, so i need help to how to write the code and implement cake php in projects.
[...] Полезные функции [...]
Hi
Could you explain how:
$this->httpGet($url)
works. Do you need to included something extra?
Jono: I think it was something custom I did. Checkout the HttpSocket class in CakePHP 1.2 for stuff like this.
Thanks Felix. Great blog by the way, really enjoying CAKE PHP and your Blog definitely helps with the documentation still not being finished.
Still relevant - some of us have no plans to upgrade and still have tweaks we could make. Thanks for keeping this online :)
Wow, thank you SO MUCH for the generateFieldNames() and $form-> generateFields(generateFieldNames()) functions....!!!
This is excellent, thanks. Didn't think it was possible for me to find anything I *didn't know already* on Cake!! Thanks Felix, your site/posts are great.
Regards,
Matt Kaufman
917-512-6281
This post is too old. We do not allow comments here anymore in order to fight spam. If you have real feedback or questions for the post, please contact us.
[...] ThinkingPHP » Yummy CakePHP functions you should know about! Some useful, tucked out of sight functions in the core CakePHP API (tags: cakephp) [...]